Faster Fourier - Bessel Coefficient Calculations on Python
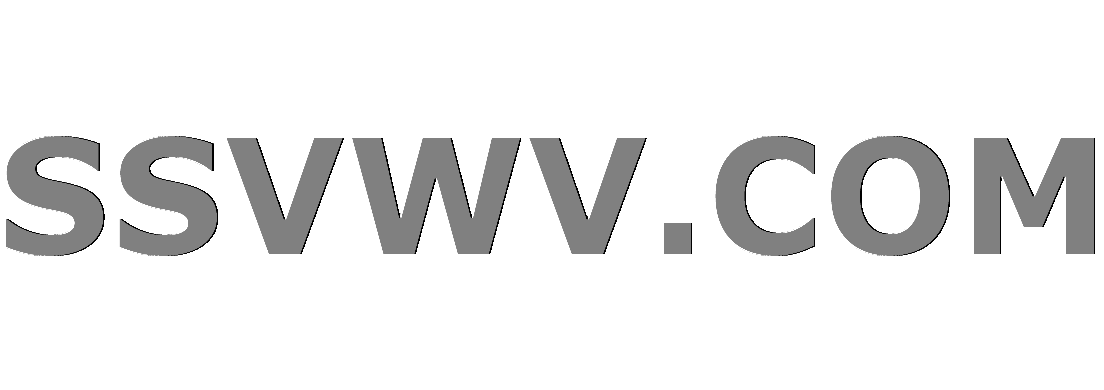
Multi tool use
Clash Royale CLAN TAG#URR8PPP
up vote
0
down vote
favorite
So I'm trying to get the fourier-bessel coefficients of a very large array of numbers that are around 1 million points in size, but I'm coming across some speed issues with calculating $J_o(x)$ for a large array.
I have my put code below (Python)
import numpy as np
import scipy.integrate as integrate
from scipy.special import j0, j1, jn_zeros
def fourier_bessel_coeffs(x, num_coeffs=None):
if num_coeffs is None:
num_coeffs = len(x)
# Roots of the Zeroth-Order Bessel Function
zeroth_roots = jn_zeros(0, num_coeffs)
# Define domain
t = np.arange(0, len(x))
# Define end point of domain, which is required as paramter
a = len(x)
# Calculate Coefficients
coeffs = np.array([2*integrate.trapz(t*x*j0(i*t/a)) / (a**2 * j1(i)**2)
for i in zeroth_roots])
return coeffs
The code is based on the following:
Any signal $y(t)$ can be represented as a fourier-bessel expansion:
beginequation
y(t) = sum_n=1^N D_nJ_0 left( fraclambda_nat right)
endequation
Where $J_0(cdot)$ are zero-order Bessel functions and the $lambda_n; n = 1,2,..., N$ is the ascending order positive roots of $J_0(lambda) = 0$
Using the orthogonality of the set $ left J_0 left( fraclambda_nat right) right$ , the FB coefficients $D_n$ are computed by the following:
beginequation
D_n = frac2 int_0^a ty(t) J_0 left( fraclambda_nat right) dta^2
endequation
with $1 leq n leq N$ and $J_1(lambda_n)$ are the first order bessel functions.
From what I've been trying out, is that it gets hung up when trying to compute $J_0(fraclambda_na t)$
Edit: Note x = $y(t)$ in the code
fourier-analysis computational-mathematics bessel-functions
 |Â
show 2 more comments
up vote
0
down vote
favorite
So I'm trying to get the fourier-bessel coefficients of a very large array of numbers that are around 1 million points in size, but I'm coming across some speed issues with calculating $J_o(x)$ for a large array.
I have my put code below (Python)
import numpy as np
import scipy.integrate as integrate
from scipy.special import j0, j1, jn_zeros
def fourier_bessel_coeffs(x, num_coeffs=None):
if num_coeffs is None:
num_coeffs = len(x)
# Roots of the Zeroth-Order Bessel Function
zeroth_roots = jn_zeros(0, num_coeffs)
# Define domain
t = np.arange(0, len(x))
# Define end point of domain, which is required as paramter
a = len(x)
# Calculate Coefficients
coeffs = np.array([2*integrate.trapz(t*x*j0(i*t/a)) / (a**2 * j1(i)**2)
for i in zeroth_roots])
return coeffs
The code is based on the following:
Any signal $y(t)$ can be represented as a fourier-bessel expansion:
beginequation
y(t) = sum_n=1^N D_nJ_0 left( fraclambda_nat right)
endequation
Where $J_0(cdot)$ are zero-order Bessel functions and the $lambda_n; n = 1,2,..., N$ is the ascending order positive roots of $J_0(lambda) = 0$
Using the orthogonality of the set $ left J_0 left( fraclambda_nat right) right$ , the FB coefficients $D_n$ are computed by the following:
beginequation
D_n = frac2 int_0^a ty(t) J_0 left( fraclambda_nat right) dta^2
endequation
with $1 leq n leq N$ and $J_1(lambda_n)$ are the first order bessel functions.
From what I've been trying out, is that it gets hung up when trying to compute $J_0(fraclambda_na t)$
Edit: Note x = $y(t)$ in the code
fourier-analysis computational-mathematics bessel-functions
Why is the number of coefficients you want the same as the length of $y(t)$?
– Winther
Aug 28 at 15:25
@Winther wouldn't I need as many coefficients as I have points of $y(t)$ to accurately represent $y(t)$
– Sam
Aug 28 at 15:26
It depends on the function how many you need, but generally I would think you would need significantly less. Just test it. Reducing this could speed it up significantly.
– Winther
Aug 28 at 15:28
Another issue with your code is that you only sample the function at integer $t$'s. So if $a=2$ then you only have $2$ points to represent it. This seems strange to me.
– Winther
Aug 28 at 15:51
@Winther fair point , though doesn't $a$ in this case represent the region of integration of the the function $y(t)$ so wouldn't $a$ always be the length of $y(t)$
– Sam
Aug 28 at 16:00
 |Â
show 2 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
So I'm trying to get the fourier-bessel coefficients of a very large array of numbers that are around 1 million points in size, but I'm coming across some speed issues with calculating $J_o(x)$ for a large array.
I have my put code below (Python)
import numpy as np
import scipy.integrate as integrate
from scipy.special import j0, j1, jn_zeros
def fourier_bessel_coeffs(x, num_coeffs=None):
if num_coeffs is None:
num_coeffs = len(x)
# Roots of the Zeroth-Order Bessel Function
zeroth_roots = jn_zeros(0, num_coeffs)
# Define domain
t = np.arange(0, len(x))
# Define end point of domain, which is required as paramter
a = len(x)
# Calculate Coefficients
coeffs = np.array([2*integrate.trapz(t*x*j0(i*t/a)) / (a**2 * j1(i)**2)
for i in zeroth_roots])
return coeffs
The code is based on the following:
Any signal $y(t)$ can be represented as a fourier-bessel expansion:
beginequation
y(t) = sum_n=1^N D_nJ_0 left( fraclambda_nat right)
endequation
Where $J_0(cdot)$ are zero-order Bessel functions and the $lambda_n; n = 1,2,..., N$ is the ascending order positive roots of $J_0(lambda) = 0$
Using the orthogonality of the set $ left J_0 left( fraclambda_nat right) right$ , the FB coefficients $D_n$ are computed by the following:
beginequation
D_n = frac2 int_0^a ty(t) J_0 left( fraclambda_nat right) dta^2
endequation
with $1 leq n leq N$ and $J_1(lambda_n)$ are the first order bessel functions.
From what I've been trying out, is that it gets hung up when trying to compute $J_0(fraclambda_na t)$
Edit: Note x = $y(t)$ in the code
fourier-analysis computational-mathematics bessel-functions
So I'm trying to get the fourier-bessel coefficients of a very large array of numbers that are around 1 million points in size, but I'm coming across some speed issues with calculating $J_o(x)$ for a large array.
I have my put code below (Python)
import numpy as np
import scipy.integrate as integrate
from scipy.special import j0, j1, jn_zeros
def fourier_bessel_coeffs(x, num_coeffs=None):
if num_coeffs is None:
num_coeffs = len(x)
# Roots of the Zeroth-Order Bessel Function
zeroth_roots = jn_zeros(0, num_coeffs)
# Define domain
t = np.arange(0, len(x))
# Define end point of domain, which is required as paramter
a = len(x)
# Calculate Coefficients
coeffs = np.array([2*integrate.trapz(t*x*j0(i*t/a)) / (a**2 * j1(i)**2)
for i in zeroth_roots])
return coeffs
The code is based on the following:
Any signal $y(t)$ can be represented as a fourier-bessel expansion:
beginequation
y(t) = sum_n=1^N D_nJ_0 left( fraclambda_nat right)
endequation
Where $J_0(cdot)$ are zero-order Bessel functions and the $lambda_n; n = 1,2,..., N$ is the ascending order positive roots of $J_0(lambda) = 0$
Using the orthogonality of the set $ left J_0 left( fraclambda_nat right) right$ , the FB coefficients $D_n$ are computed by the following:
beginequation
D_n = frac2 int_0^a ty(t) J_0 left( fraclambda_nat right) dta^2
endequation
with $1 leq n leq N$ and $J_1(lambda_n)$ are the first order bessel functions.
From what I've been trying out, is that it gets hung up when trying to compute $J_0(fraclambda_na t)$
Edit: Note x = $y(t)$ in the code
fourier-analysis computational-mathematics bessel-functions
asked Aug 28 at 15:01
Sam
164
164
Why is the number of coefficients you want the same as the length of $y(t)$?
– Winther
Aug 28 at 15:25
@Winther wouldn't I need as many coefficients as I have points of $y(t)$ to accurately represent $y(t)$
– Sam
Aug 28 at 15:26
It depends on the function how many you need, but generally I would think you would need significantly less. Just test it. Reducing this could speed it up significantly.
– Winther
Aug 28 at 15:28
Another issue with your code is that you only sample the function at integer $t$'s. So if $a=2$ then you only have $2$ points to represent it. This seems strange to me.
– Winther
Aug 28 at 15:51
@Winther fair point , though doesn't $a$ in this case represent the region of integration of the the function $y(t)$ so wouldn't $a$ always be the length of $y(t)$
– Sam
Aug 28 at 16:00
 |Â
show 2 more comments
Why is the number of coefficients you want the same as the length of $y(t)$?
– Winther
Aug 28 at 15:25
@Winther wouldn't I need as many coefficients as I have points of $y(t)$ to accurately represent $y(t)$
– Sam
Aug 28 at 15:26
It depends on the function how many you need, but generally I would think you would need significantly less. Just test it. Reducing this could speed it up significantly.
– Winther
Aug 28 at 15:28
Another issue with your code is that you only sample the function at integer $t$'s. So if $a=2$ then you only have $2$ points to represent it. This seems strange to me.
– Winther
Aug 28 at 15:51
@Winther fair point , though doesn't $a$ in this case represent the region of integration of the the function $y(t)$ so wouldn't $a$ always be the length of $y(t)$
– Sam
Aug 28 at 16:00
Why is the number of coefficients you want the same as the length of $y(t)$?
– Winther
Aug 28 at 15:25
Why is the number of coefficients you want the same as the length of $y(t)$?
– Winther
Aug 28 at 15:25
@Winther wouldn't I need as many coefficients as I have points of $y(t)$ to accurately represent $y(t)$
– Sam
Aug 28 at 15:26
@Winther wouldn't I need as many coefficients as I have points of $y(t)$ to accurately represent $y(t)$
– Sam
Aug 28 at 15:26
It depends on the function how many you need, but generally I would think you would need significantly less. Just test it. Reducing this could speed it up significantly.
– Winther
Aug 28 at 15:28
It depends on the function how many you need, but generally I would think you would need significantly less. Just test it. Reducing this could speed it up significantly.
– Winther
Aug 28 at 15:28
Another issue with your code is that you only sample the function at integer $t$'s. So if $a=2$ then you only have $2$ points to represent it. This seems strange to me.
– Winther
Aug 28 at 15:51
Another issue with your code is that you only sample the function at integer $t$'s. So if $a=2$ then you only have $2$ points to represent it. This seems strange to me.
– Winther
Aug 28 at 15:51
@Winther fair point , though doesn't $a$ in this case represent the region of integration of the the function $y(t)$ so wouldn't $a$ always be the length of $y(t)$
– Sam
Aug 28 at 16:00
@Winther fair point , though doesn't $a$ in this case represent the region of integration of the the function $y(t)$ so wouldn't $a$ always be the length of $y(t)$
– Sam
Aug 28 at 16:00
 |Â
show 2 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmath.stackexchange.com%2fquestions%2f2897367%2ffaster-fourier-bessel-coefficient-calculations-on-python%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Why is the number of coefficients you want the same as the length of $y(t)$?
– Winther
Aug 28 at 15:25
@Winther wouldn't I need as many coefficients as I have points of $y(t)$ to accurately represent $y(t)$
– Sam
Aug 28 at 15:26
It depends on the function how many you need, but generally I would think you would need significantly less. Just test it. Reducing this could speed it up significantly.
– Winther
Aug 28 at 15:28
Another issue with your code is that you only sample the function at integer $t$'s. So if $a=2$ then you only have $2$ points to represent it. This seems strange to me.
– Winther
Aug 28 at 15:51
@Winther fair point , though doesn't $a$ in this case represent the region of integration of the the function $y(t)$ so wouldn't $a$ always be the length of $y(t)$
– Sam
Aug 28 at 16:00