get the points of a hexagon knowing only a what length each side should be and a point
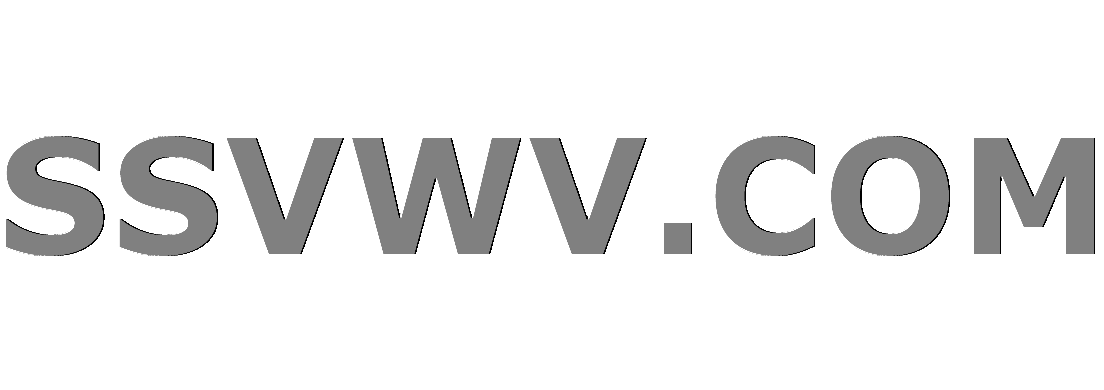
Multi tool use
Clash Royale CLAN TAG#URR8PPP
up vote
0
down vote
favorite
I am trying to write a program to display a hexagon. The only values I know are a starting point, e.g. 0, 0
How can I know the other 5 points if I want each
length to be 2 units long for example.
The interior angle of a hexagon is 120%.
But I am struggling to know how to get the next points from the starting position and knowing the above facts.
I have found this code:
export const createPoints = (startingPoint: Point) =>
const _s32 = Math.sqrt(3) / 2;
const A = 25;
const xDiff = 100;
const yDiff = 100;
return [
[A + xDiff, 0 + yDiff],
[A / 2 + xDiff, A * _s32 + yDiff],
[-A / 2 + xDiff, A * _s32 + yDiff],
[-A + xDiff, 0 + yDiff],
[-A / 2 + xDiff, -A * _s32 + yDiff],
[A / 2 + xDiff, -A * _s32 + yDiff]
];
;
But I do not understand the calculation.
_s32
is the square root of 3 divided by two
trigonometry
add a comment |Â
up vote
0
down vote
favorite
I am trying to write a program to display a hexagon. The only values I know are a starting point, e.g. 0, 0
How can I know the other 5 points if I want each
length to be 2 units long for example.
The interior angle of a hexagon is 120%.
But I am struggling to know how to get the next points from the starting position and knowing the above facts.
I have found this code:
export const createPoints = (startingPoint: Point) =>
const _s32 = Math.sqrt(3) / 2;
const A = 25;
const xDiff = 100;
const yDiff = 100;
return [
[A + xDiff, 0 + yDiff],
[A / 2 + xDiff, A * _s32 + yDiff],
[-A / 2 + xDiff, A * _s32 + yDiff],
[-A + xDiff, 0 + yDiff],
[-A / 2 + xDiff, -A * _s32 + yDiff],
[A / 2 + xDiff, -A * _s32 + yDiff]
];
;
But I do not understand the calculation.
_s32
is the square root of 3 divided by two
trigonometry
1
These values do not determine the hexagon uniquely. Rotations around (0,0) are not prevented. Also think about reflections
– daw
Sep 4 at 12:26
add a comment |Â
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to write a program to display a hexagon. The only values I know are a starting point, e.g. 0, 0
How can I know the other 5 points if I want each
length to be 2 units long for example.
The interior angle of a hexagon is 120%.
But I am struggling to know how to get the next points from the starting position and knowing the above facts.
I have found this code:
export const createPoints = (startingPoint: Point) =>
const _s32 = Math.sqrt(3) / 2;
const A = 25;
const xDiff = 100;
const yDiff = 100;
return [
[A + xDiff, 0 + yDiff],
[A / 2 + xDiff, A * _s32 + yDiff],
[-A / 2 + xDiff, A * _s32 + yDiff],
[-A + xDiff, 0 + yDiff],
[-A / 2 + xDiff, -A * _s32 + yDiff],
[A / 2 + xDiff, -A * _s32 + yDiff]
];
;
But I do not understand the calculation.
_s32
is the square root of 3 divided by two
trigonometry
I am trying to write a program to display a hexagon. The only values I know are a starting point, e.g. 0, 0
How can I know the other 5 points if I want each
length to be 2 units long for example.
The interior angle of a hexagon is 120%.
But I am struggling to know how to get the next points from the starting position and knowing the above facts.
I have found this code:
export const createPoints = (startingPoint: Point) =>
const _s32 = Math.sqrt(3) / 2;
const A = 25;
const xDiff = 100;
const yDiff = 100;
return [
[A + xDiff, 0 + yDiff],
[A / 2 + xDiff, A * _s32 + yDiff],
[-A / 2 + xDiff, A * _s32 + yDiff],
[-A + xDiff, 0 + yDiff],
[-A / 2 + xDiff, -A * _s32 + yDiff],
[A / 2 + xDiff, -A * _s32 + yDiff]
];
;
But I do not understand the calculation.
_s32
is the square root of 3 divided by two
trigonometry
trigonometry
edited Sep 4 at 12:49
asked Sep 4 at 12:19
dagda1
230923
230923
1
These values do not determine the hexagon uniquely. Rotations around (0,0) are not prevented. Also think about reflections
– daw
Sep 4 at 12:26
add a comment |Â
1
These values do not determine the hexagon uniquely. Rotations around (0,0) are not prevented. Also think about reflections
– daw
Sep 4 at 12:26
1
1
These values do not determine the hexagon uniquely. Rotations around (0,0) are not prevented. Also think about reflections
– daw
Sep 4 at 12:26
These values do not determine the hexagon uniquely. Rotations around (0,0) are not prevented. Also think about reflections
– daw
Sep 4 at 12:26
add a comment |Â
1 Answer
1
active
oldest
votes
up vote
0
down vote
The trick is to rotate the hexagon around the center of its mass, then you just need to rotate the radius by 60 degrees to get each point. Here's a pseudo code
p0 = (0, 0) # location of the center - free parameter
phi = 0.0 # angle of the 1st point w.r.t x axis - free parameter
r = 10.0 # radius of the hexagon - free parameter
p1 = p0 + r*(cos(0*60 + phi), sin(0*60 + phi))
p2 = p0 + r*(cos(1*60 + phi), sin(1*60 + phi))
p3 = p0 + r*(cos(2*60 + phi), sin(2*60 + phi))
p4 = p0 + r*(cos(3*60 + phi), sin(3*60 + phi))
p5 = p0 + r*(cos(4*60 + phi), sin(4*60 + phi))
p6 = p0 + r*(cos(5*60 + phi), sin(5*60 + phi))
Note I have written all angles in degrees - you will want to convert them to radians multiplying them by $pi / 180$
add a comment |Â
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The trick is to rotate the hexagon around the center of its mass, then you just need to rotate the radius by 60 degrees to get each point. Here's a pseudo code
p0 = (0, 0) # location of the center - free parameter
phi = 0.0 # angle of the 1st point w.r.t x axis - free parameter
r = 10.0 # radius of the hexagon - free parameter
p1 = p0 + r*(cos(0*60 + phi), sin(0*60 + phi))
p2 = p0 + r*(cos(1*60 + phi), sin(1*60 + phi))
p3 = p0 + r*(cos(2*60 + phi), sin(2*60 + phi))
p4 = p0 + r*(cos(3*60 + phi), sin(3*60 + phi))
p5 = p0 + r*(cos(4*60 + phi), sin(4*60 + phi))
p6 = p0 + r*(cos(5*60 + phi), sin(5*60 + phi))
Note I have written all angles in degrees - you will want to convert them to radians multiplying them by $pi / 180$
add a comment |Â
up vote
0
down vote
The trick is to rotate the hexagon around the center of its mass, then you just need to rotate the radius by 60 degrees to get each point. Here's a pseudo code
p0 = (0, 0) # location of the center - free parameter
phi = 0.0 # angle of the 1st point w.r.t x axis - free parameter
r = 10.0 # radius of the hexagon - free parameter
p1 = p0 + r*(cos(0*60 + phi), sin(0*60 + phi))
p2 = p0 + r*(cos(1*60 + phi), sin(1*60 + phi))
p3 = p0 + r*(cos(2*60 + phi), sin(2*60 + phi))
p4 = p0 + r*(cos(3*60 + phi), sin(3*60 + phi))
p5 = p0 + r*(cos(4*60 + phi), sin(4*60 + phi))
p6 = p0 + r*(cos(5*60 + phi), sin(5*60 + phi))
Note I have written all angles in degrees - you will want to convert them to radians multiplying them by $pi / 180$
add a comment |Â
up vote
0
down vote
up vote
0
down vote
The trick is to rotate the hexagon around the center of its mass, then you just need to rotate the radius by 60 degrees to get each point. Here's a pseudo code
p0 = (0, 0) # location of the center - free parameter
phi = 0.0 # angle of the 1st point w.r.t x axis - free parameter
r = 10.0 # radius of the hexagon - free parameter
p1 = p0 + r*(cos(0*60 + phi), sin(0*60 + phi))
p2 = p0 + r*(cos(1*60 + phi), sin(1*60 + phi))
p3 = p0 + r*(cos(2*60 + phi), sin(2*60 + phi))
p4 = p0 + r*(cos(3*60 + phi), sin(3*60 + phi))
p5 = p0 + r*(cos(4*60 + phi), sin(4*60 + phi))
p6 = p0 + r*(cos(5*60 + phi), sin(5*60 + phi))
Note I have written all angles in degrees - you will want to convert them to radians multiplying them by $pi / 180$
The trick is to rotate the hexagon around the center of its mass, then you just need to rotate the radius by 60 degrees to get each point. Here's a pseudo code
p0 = (0, 0) # location of the center - free parameter
phi = 0.0 # angle of the 1st point w.r.t x axis - free parameter
r = 10.0 # radius of the hexagon - free parameter
p1 = p0 + r*(cos(0*60 + phi), sin(0*60 + phi))
p2 = p0 + r*(cos(1*60 + phi), sin(1*60 + phi))
p3 = p0 + r*(cos(2*60 + phi), sin(2*60 + phi))
p4 = p0 + r*(cos(3*60 + phi), sin(3*60 + phi))
p5 = p0 + r*(cos(4*60 + phi), sin(4*60 + phi))
p6 = p0 + r*(cos(5*60 + phi), sin(5*60 + phi))
Note I have written all angles in degrees - you will want to convert them to radians multiplying them by $pi / 180$
answered Sep 4 at 13:00


Aleksejs Fomins
388111
388111
add a comment |Â
add a comment |Â
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmath.stackexchange.com%2fquestions%2f2904985%2fget-the-points-of-a-hexagon-knowing-only-a-what-length-each-side-should-be-and-a%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
1
These values do not determine the hexagon uniquely. Rotations around (0,0) are not prevented. Also think about reflections
– daw
Sep 4 at 12:26