Interactive Scatter plot in R using Plotly and Shiny
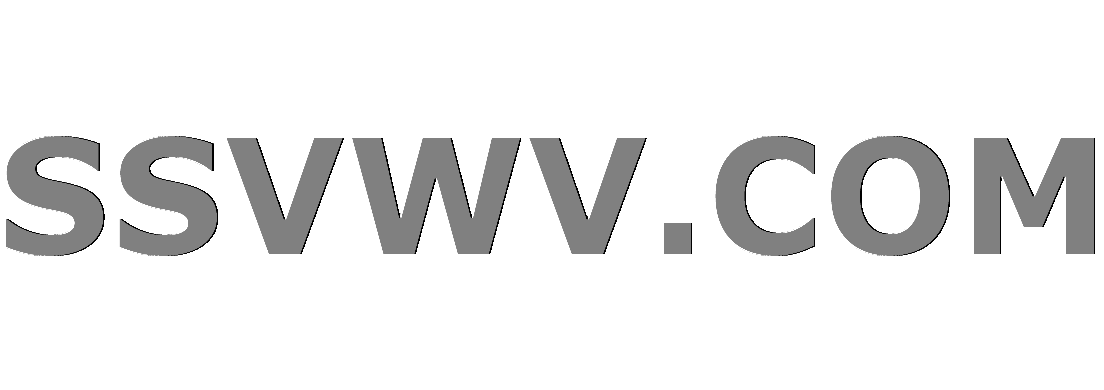
Multi tool use
up vote
1
down vote
favorite
I am relatively new to Shiny and Plotly and have the following code snippet:
#
# This is a Shiny web application. You can run the application by clicking
# the 'Run App' button above.
#
# Find out more about building applications with Shiny here:
#
# http://shiny.rstudio.com/
#
library(shiny)
library(plotly)
library(odbc)
library(DBI)
# Define UI for application that draws a histogram
ui <- fluidPage(
# Application title
titlePanel("Demo"),
#Sidebar with a slider input for number of bins
sidebarLayout(
sidebarPanel(
sliderInput("bins",
"Number of bins:",
min = 0,
max = 100,
value = 70)
),
# Show a plot of the generated distribution
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"))
)
)
)
# Define server logic required to draw a histogram
server <- function(input, output, session)
QueriedData <- reactive(
connn <- DBI::dbConnect(odbc::odbc(),.connection_string = "XXX", uid = "AB", pwd = "CD")
lat_rec.df <- dbGetQuery(connn, "PQR")
dbDisconnect(connn)
lat_rec.df1
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(),x = ~TotalCount, y = ~MyScore, type = 'scatter', mode = 'markers')
)
# Run the application
shinyApp(ui = ui, server = server)
As you can see above, I am plotting a scatter plot of my dataframe which I have read from the Database (as mentioned in the reactive function). I have a couple of questions here:
- I want to use the slider bar input as my Y Axis (MyScore). How do I do that? I am currently unable to link slider bar (bins) into my plotly graph. I want the scatter plot to update as per the slider input.
- I am slightly confused about reactive functions. Does it mean that each time, I change the slider bar, the DB is going to get called (in reactive function)? How does it work?
- If I have other Database tables to read and plot in other areas, do I include it in the reactive function? Please advice.
Thanks in advance for all your help! Cheers!
r shiny r-plotly
add a comment |
up vote
1
down vote
favorite
I am relatively new to Shiny and Plotly and have the following code snippet:
#
# This is a Shiny web application. You can run the application by clicking
# the 'Run App' button above.
#
# Find out more about building applications with Shiny here:
#
# http://shiny.rstudio.com/
#
library(shiny)
library(plotly)
library(odbc)
library(DBI)
# Define UI for application that draws a histogram
ui <- fluidPage(
# Application title
titlePanel("Demo"),
#Sidebar with a slider input for number of bins
sidebarLayout(
sidebarPanel(
sliderInput("bins",
"Number of bins:",
min = 0,
max = 100,
value = 70)
),
# Show a plot of the generated distribution
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"))
)
)
)
# Define server logic required to draw a histogram
server <- function(input, output, session)
QueriedData <- reactive(
connn <- DBI::dbConnect(odbc::odbc(),.connection_string = "XXX", uid = "AB", pwd = "CD")
lat_rec.df <- dbGetQuery(connn, "PQR")
dbDisconnect(connn)
lat_rec.df1
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(),x = ~TotalCount, y = ~MyScore, type = 'scatter', mode = 'markers')
)
# Run the application
shinyApp(ui = ui, server = server)
As you can see above, I am plotting a scatter plot of my dataframe which I have read from the Database (as mentioned in the reactive function). I have a couple of questions here:
- I want to use the slider bar input as my Y Axis (MyScore). How do I do that? I am currently unable to link slider bar (bins) into my plotly graph. I want the scatter plot to update as per the slider input.
- I am slightly confused about reactive functions. Does it mean that each time, I change the slider bar, the DB is going to get called (in reactive function)? How does it work?
- If I have other Database tables to read and plot in other areas, do I include it in the reactive function? Please advice.
Thanks in advance for all your help! Cheers!
r shiny r-plotly
need some more code to be added for scatter plot with ui and server finally u need to renderplot to be used. i have saw few articles in shiny pages only.
– sai saran
23 hours ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am relatively new to Shiny and Plotly and have the following code snippet:
#
# This is a Shiny web application. You can run the application by clicking
# the 'Run App' button above.
#
# Find out more about building applications with Shiny here:
#
# http://shiny.rstudio.com/
#
library(shiny)
library(plotly)
library(odbc)
library(DBI)
# Define UI for application that draws a histogram
ui <- fluidPage(
# Application title
titlePanel("Demo"),
#Sidebar with a slider input for number of bins
sidebarLayout(
sidebarPanel(
sliderInput("bins",
"Number of bins:",
min = 0,
max = 100,
value = 70)
),
# Show a plot of the generated distribution
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"))
)
)
)
# Define server logic required to draw a histogram
server <- function(input, output, session)
QueriedData <- reactive(
connn <- DBI::dbConnect(odbc::odbc(),.connection_string = "XXX", uid = "AB", pwd = "CD")
lat_rec.df <- dbGetQuery(connn, "PQR")
dbDisconnect(connn)
lat_rec.df1
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(),x = ~TotalCount, y = ~MyScore, type = 'scatter', mode = 'markers')
)
# Run the application
shinyApp(ui = ui, server = server)
As you can see above, I am plotting a scatter plot of my dataframe which I have read from the Database (as mentioned in the reactive function). I have a couple of questions here:
- I want to use the slider bar input as my Y Axis (MyScore). How do I do that? I am currently unable to link slider bar (bins) into my plotly graph. I want the scatter plot to update as per the slider input.
- I am slightly confused about reactive functions. Does it mean that each time, I change the slider bar, the DB is going to get called (in reactive function)? How does it work?
- If I have other Database tables to read and plot in other areas, do I include it in the reactive function? Please advice.
Thanks in advance for all your help! Cheers!
r shiny r-plotly
I am relatively new to Shiny and Plotly and have the following code snippet:
#
# This is a Shiny web application. You can run the application by clicking
# the 'Run App' button above.
#
# Find out more about building applications with Shiny here:
#
# http://shiny.rstudio.com/
#
library(shiny)
library(plotly)
library(odbc)
library(DBI)
# Define UI for application that draws a histogram
ui <- fluidPage(
# Application title
titlePanel("Demo"),
#Sidebar with a slider input for number of bins
sidebarLayout(
sidebarPanel(
sliderInput("bins",
"Number of bins:",
min = 0,
max = 100,
value = 70)
),
# Show a plot of the generated distribution
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"))
)
)
)
# Define server logic required to draw a histogram
server <- function(input, output, session)
QueriedData <- reactive(
connn <- DBI::dbConnect(odbc::odbc(),.connection_string = "XXX", uid = "AB", pwd = "CD")
lat_rec.df <- dbGetQuery(connn, "PQR")
dbDisconnect(connn)
lat_rec.df1
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(),x = ~TotalCount, y = ~MyScore, type = 'scatter', mode = 'markers')
)
# Run the application
shinyApp(ui = ui, server = server)
As you can see above, I am plotting a scatter plot of my dataframe which I have read from the Database (as mentioned in the reactive function). I have a couple of questions here:
- I want to use the slider bar input as my Y Axis (MyScore). How do I do that? I am currently unable to link slider bar (bins) into my plotly graph. I want the scatter plot to update as per the slider input.
- I am slightly confused about reactive functions. Does it mean that each time, I change the slider bar, the DB is going to get called (in reactive function)? How does it work?
- If I have other Database tables to read and plot in other areas, do I include it in the reactive function? Please advice.
Thanks in advance for all your help! Cheers!
r shiny r-plotly
r shiny r-plotly
asked yesterday
ruser
395
395
need some more code to be added for scatter plot with ui and server finally u need to renderplot to be used. i have saw few articles in shiny pages only.
– sai saran
23 hours ago
add a comment |
need some more code to be added for scatter plot with ui and server finally u need to renderplot to be used. i have saw few articles in shiny pages only.
– sai saran
23 hours ago
need some more code to be added for scatter plot with ui and server finally u need to renderplot to be used. i have saw few articles in shiny pages only.
– sai saran
23 hours ago
need some more code to be added for scatter plot with ui and server finally u need to renderplot to be used. i have saw few articles in shiny pages only.
– sai saran
23 hours ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
My solutions/answers to your three questions.
1.As you want to know how to control Y axis with sliderInput below code explains how to do it.
library(shiny)
library(plotly)
library(DBI)
library(pool)
pool <- dbPool(drv = RMySQL::MySQL(),dbname = "db",host = "localhost",username = "root",password = "psw", port = 3306)
data <- dbGetQuery(pool, "SELECT * FROM testTable;")
ui <- fluidPage(
titlePanel("Demo"),
sidebarLayout(
sidebarPanel(
sliderInput("bins", "Number of bins:", min = 0, max = 100, value = 70)
),
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"),
plotOutput("basicPlot") # Added extra as an Example for 3rd question
)
)
)
)
server <- function(input, output, session)
QueriedData <- reactive(
df <- data[data$total <= input$bins,] # filtering datafarme based on sliderInput
return(df)
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(), x = ~count, y = ~total, type = 'scatter', mode = 'markers')
)
# Added extra as an Example for 3rd question
output$basicPlot <- renderPlot(
data_for_plot <- dbGetQuery(pool, "SELECT * FROM dummyTable WHERE uid = 2018;")
plot(x = data_for_plot$category, y = data_for_plot$performance, type = "p")
)
shinyApp(ui = ui, server = server)
2.For reactivity it is better to fetch the table into a dataframe once, then place that dataframe in reactive environment. So that you can avoid multiple database calls. You can check in above code for the same.
3.Using of reactive
environment purely depends on the requirement when you want to have interactivity with your shiny application. If you want to fetch the data from other tables and use in different plots, then no need to place your database connection string in reactive environment. Just query the database according to your requirement like in above code.
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
1
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
My solutions/answers to your three questions.
1.As you want to know how to control Y axis with sliderInput below code explains how to do it.
library(shiny)
library(plotly)
library(DBI)
library(pool)
pool <- dbPool(drv = RMySQL::MySQL(),dbname = "db",host = "localhost",username = "root",password = "psw", port = 3306)
data <- dbGetQuery(pool, "SELECT * FROM testTable;")
ui <- fluidPage(
titlePanel("Demo"),
sidebarLayout(
sidebarPanel(
sliderInput("bins", "Number of bins:", min = 0, max = 100, value = 70)
),
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"),
plotOutput("basicPlot") # Added extra as an Example for 3rd question
)
)
)
)
server <- function(input, output, session)
QueriedData <- reactive(
df <- data[data$total <= input$bins,] # filtering datafarme based on sliderInput
return(df)
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(), x = ~count, y = ~total, type = 'scatter', mode = 'markers')
)
# Added extra as an Example for 3rd question
output$basicPlot <- renderPlot(
data_for_plot <- dbGetQuery(pool, "SELECT * FROM dummyTable WHERE uid = 2018;")
plot(x = data_for_plot$category, y = data_for_plot$performance, type = "p")
)
shinyApp(ui = ui, server = server)
2.For reactivity it is better to fetch the table into a dataframe once, then place that dataframe in reactive environment. So that you can avoid multiple database calls. You can check in above code for the same.
3.Using of reactive
environment purely depends on the requirement when you want to have interactivity with your shiny application. If you want to fetch the data from other tables and use in different plots, then no need to place your database connection string in reactive environment. Just query the database according to your requirement like in above code.
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
1
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
add a comment |
up vote
1
down vote
accepted
My solutions/answers to your three questions.
1.As you want to know how to control Y axis with sliderInput below code explains how to do it.
library(shiny)
library(plotly)
library(DBI)
library(pool)
pool <- dbPool(drv = RMySQL::MySQL(),dbname = "db",host = "localhost",username = "root",password = "psw", port = 3306)
data <- dbGetQuery(pool, "SELECT * FROM testTable;")
ui <- fluidPage(
titlePanel("Demo"),
sidebarLayout(
sidebarPanel(
sliderInput("bins", "Number of bins:", min = 0, max = 100, value = 70)
),
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"),
plotOutput("basicPlot") # Added extra as an Example for 3rd question
)
)
)
)
server <- function(input, output, session)
QueriedData <- reactive(
df <- data[data$total <= input$bins,] # filtering datafarme based on sliderInput
return(df)
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(), x = ~count, y = ~total, type = 'scatter', mode = 'markers')
)
# Added extra as an Example for 3rd question
output$basicPlot <- renderPlot(
data_for_plot <- dbGetQuery(pool, "SELECT * FROM dummyTable WHERE uid = 2018;")
plot(x = data_for_plot$category, y = data_for_plot$performance, type = "p")
)
shinyApp(ui = ui, server = server)
2.For reactivity it is better to fetch the table into a dataframe once, then place that dataframe in reactive environment. So that you can avoid multiple database calls. You can check in above code for the same.
3.Using of reactive
environment purely depends on the requirement when you want to have interactivity with your shiny application. If you want to fetch the data from other tables and use in different plots, then no need to place your database connection string in reactive environment. Just query the database according to your requirement like in above code.
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
1
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
My solutions/answers to your three questions.
1.As you want to know how to control Y axis with sliderInput below code explains how to do it.
library(shiny)
library(plotly)
library(DBI)
library(pool)
pool <- dbPool(drv = RMySQL::MySQL(),dbname = "db",host = "localhost",username = "root",password = "psw", port = 3306)
data <- dbGetQuery(pool, "SELECT * FROM testTable;")
ui <- fluidPage(
titlePanel("Demo"),
sidebarLayout(
sidebarPanel(
sliderInput("bins", "Number of bins:", min = 0, max = 100, value = 70)
),
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"),
plotOutput("basicPlot") # Added extra as an Example for 3rd question
)
)
)
)
server <- function(input, output, session)
QueriedData <- reactive(
df <- data[data$total <= input$bins,] # filtering datafarme based on sliderInput
return(df)
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(), x = ~count, y = ~total, type = 'scatter', mode = 'markers')
)
# Added extra as an Example for 3rd question
output$basicPlot <- renderPlot(
data_for_plot <- dbGetQuery(pool, "SELECT * FROM dummyTable WHERE uid = 2018;")
plot(x = data_for_plot$category, y = data_for_plot$performance, type = "p")
)
shinyApp(ui = ui, server = server)
2.For reactivity it is better to fetch the table into a dataframe once, then place that dataframe in reactive environment. So that you can avoid multiple database calls. You can check in above code for the same.
3.Using of reactive
environment purely depends on the requirement when you want to have interactivity with your shiny application. If you want to fetch the data from other tables and use in different plots, then no need to place your database connection string in reactive environment. Just query the database according to your requirement like in above code.
My solutions/answers to your three questions.
1.As you want to know how to control Y axis with sliderInput below code explains how to do it.
library(shiny)
library(plotly)
library(DBI)
library(pool)
pool <- dbPool(drv = RMySQL::MySQL(),dbname = "db",host = "localhost",username = "root",password = "psw", port = 3306)
data <- dbGetQuery(pool, "SELECT * FROM testTable;")
ui <- fluidPage(
titlePanel("Demo"),
sidebarLayout(
sidebarPanel(
sliderInput("bins", "Number of bins:", min = 0, max = 100, value = 70)
),
mainPanel(
tabPanel("Heading", plotlyOutput("tbTable"),
plotOutput("basicPlot") # Added extra as an Example for 3rd question
)
)
)
)
server <- function(input, output, session)
QueriedData <- reactive(
df <- data[data$total <= input$bins,] # filtering datafarme based on sliderInput
return(df)
)
output$tbTable <- renderPlotly(
plot_ly(QueriedData(), x = ~count, y = ~total, type = 'scatter', mode = 'markers')
)
# Added extra as an Example for 3rd question
output$basicPlot <- renderPlot(
data_for_plot <- dbGetQuery(pool, "SELECT * FROM dummyTable WHERE uid = 2018;")
plot(x = data_for_plot$category, y = data_for_plot$performance, type = "p")
)
shinyApp(ui = ui, server = server)
2.For reactivity it is better to fetch the table into a dataframe once, then place that dataframe in reactive environment. So that you can avoid multiple database calls. You can check in above code for the same.
3.Using of reactive
environment purely depends on the requirement when you want to have interactivity with your shiny application. If you want to fetch the data from other tables and use in different plots, then no need to place your database connection string in reactive environment. Just query the database according to your requirement like in above code.
answered 23 hours ago


msr_003
3671315
3671315
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
1
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
add a comment |
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
1
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
Thank you so much for your code. It is really helpful and your explanation is precise as well. I have another follow up question that I am hoping you can answer as well. You see "TotalCount" and "MyScore" are 2 columns in my dataframe and the values in "MyScore" can extend from 0 to 100. In the scatter plot, I actually (now I realize) that I want to control the range of values shown taken from input slider. So in the code, I have made the following change: value = c(0,100) for slider. But I need to get that range displayed in my scatter plot. I hope you can understand my question. Thanks again
– ruser
23 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
I hope I was able to make sense; I want to display "MyScore" column in Y Axis but the range has to be decided by the Input slider bar. Thanks again and sorry for the inconvenience.
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
ok, I was able to figure it out: In the reactive function, I added the following code and got the range I wanted: df <- subset(lat_rec.df1, lat_rec.df1$MyScore <= input$bins[2] & lat_rec.df1$MyScore >= input$bins[1])
– ruser
22 hours ago
1
1
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
Glad it helped you a bit. It is nice feeling that you got solution for your followup question.
– msr_003
22 hours ago
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53222188%2finteractive-scatter-plot-in-r-using-plotly-and-shiny%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
olJj6 7WgSh7 a008sb6C,3n XmCJ,gy8qJT nO0i5DBOJP1VQ4gRg,2jwRfSqKszio8OYdt 8C2KaAVE8fUPO1d
need some more code to be added for scatter plot with ui and server finally u need to renderplot to be used. i have saw few articles in shiny pages only.
– sai saran
23 hours ago